Many of the tweets I have liked on Twitter hold useful, fun, unusual, or interesting tidbits of content. Sometimes I will want to rediscover something I liked, it may even be from over a year ago, and I wanted a better way than endlessly scrolling through to find them again.
I setup a small Node.js project that uses twitter-api-v2 to consume the Twitter API and get tweets in a JSON format.
{
"id": "23089413610",
"text": "Character is cultivated behavior.",
"userId": "13463562",
"userName": "Tyler Marés",
"userHandle": "tylermares"
}
I also built a simple UI to view the tweets, with Solid JS and Vite, using TypeScript.
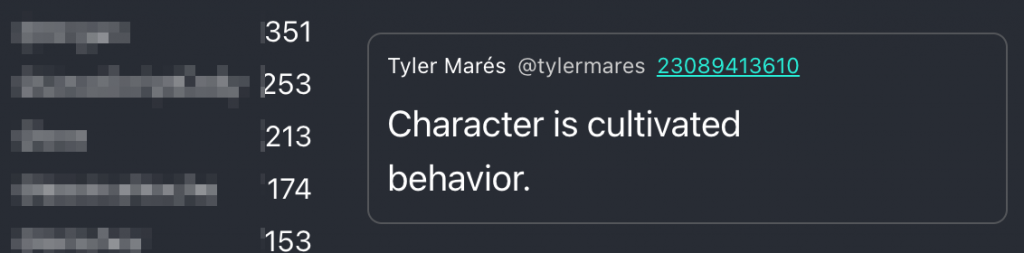
From there, I could find what I was looking for when the occasion arises in a much better way.
My favorite part of building this was implementing a way to work within API rate limits. This is a typical situation with consuming APIs, one I had come across in the Spotify Top Tracks Playlist Generator as well.
Using await syntax and a simple sleep function make it clear and concise. There’s more to it in the real project (error handling), but it goes a little something like this:
function sleep (ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
const rateUnit = 900; // 15 minutes, in seconds, before ms conversion
const timeTolerance = 500; // extra time for safety, in ms
// 75 requests per unit
const rateLimit = ((rateUnit/75) * 1000) + timeTolerance;
for await (const id of ids) {
const detail = await getDetailById(id);
formatDetail(detail); // do something with detail
await sleep(rateLimit); // honor the rate limit
}
There’s also an optimization opportunity here. Let’s say the work between requests was time consuming, in this case represented by formatDetail, but on average not longer than the wait time. Measure the elapsed time to process formatDetail, subtract it from rateLimit, and conditionally call sleep if the difference is greater than zero. No process time wasted or unaccounted for.
const detail = await getDetailById(id);
const then = performance.now();
formatDetail(detail);
const waitTime = rateLimit - (performance.now() - then);
// wait only as necessary
if (waitTime > 0) {
await sleep(waitTime);
}